How to send emojis in an email subject and body using PHP
In this post, you will learn how to send emoji or Unicode characters in the email subject and body using the PHP programming language.
There are thousands of emojis contained in Unicode 12.0. It enables us to write less and more effectively in nonverbal communication. It gives a range of emotions, feelings, anger, and happiness in small icons, and using this, we can very well communicate over mail, chat, message, comment, and so on.
The importance of emojis in email subject
According to the survey, the email open rate for the subject with emojis is 60% higher than the subject with plain text. These results show that the email with emojis is highly effective. These days, this is the key to email marketing.
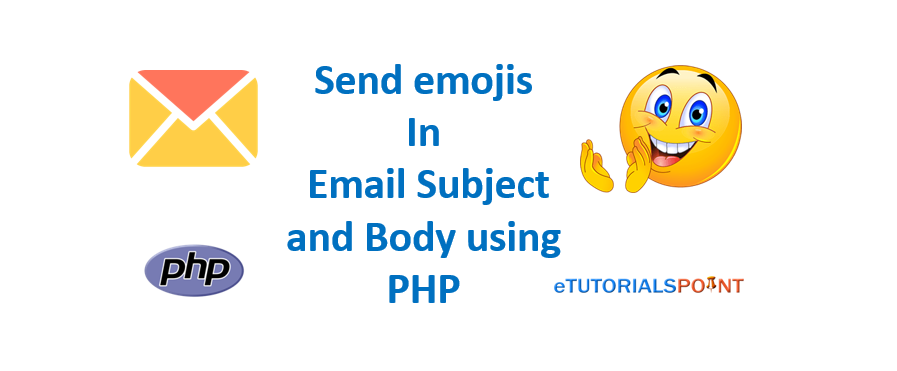
To add an emoji picker to the input field, we need emoji picker libraries. There are several emoji picker libraries available. In this example, we have taken this library from github. You can download it from here-
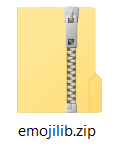
PHP Insert an emoji
To invoke the emoji picker in the input field, add (data-emojiable="true" data-emoji-input="unicode") attributes in the input element and enable the picker using the JS code.
$(function() {
window.emojiPicker = new EmojiPicker({
emojiable_selector: '[data-emojiable=true]',
assetsPath: 'emojilib/img/',
popupButtonClasses: 'fa fa-smile-o'
});
window.emojiPicker.discover();
});
Here is the main PHP file 'index.php' that we will call in the browser. We have created a form that contains four fields to enter your name, email, subject, and comment. In the head section of HTML, we have included the bootstrap CSS and emoji picker CSS files. Make sure the library path is correct. All the jQuery files from the library are added at the end of the body section. The subject and comment fields contain attributes to pick the emoji.
index.php
<?php include('sendemail.php'); ?>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0-alpha.6/css/bootstrap.min.css">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/font-awesome/4.4.0/css/font-awesome.min.css">
<link href="/emojilib/css/emoji.css" rel="stylesheet">
</head>
<body>
<form action="#" method="post">
<div class="container">
<div class="form-group">
<label for="usr">Name:</label>
<input type="text" class="form-control" id="usr" name="name">
</div>
<div class="form-group">
<label for="email">Email:</label>
<input type="text" class="form-control" id="email" name="email">
</div>
<div class="form-group">
<label for="sub">Subject:</label>
<p class="lead emoji-picker-container">
<input type="text" class="form-control" id="sub" name="subject" data-emojiable="true" data-emoji-input="unicode">
</p>
</div>
<div class="form-group">
<label for="comment">Comment:</label>
<p class="lead emoji-picker-container">
<textarea class="form-control" rows="1" data-emojiable="true" data-emoji-input="unicode" name="comment"></textarea>
</p>
</div>
<div class="form-group">
<input type="submit" class="btn btn-info" value="Submit">
</div>
</div>
</form>
<script src="https://code.jquery.com/jquery-1.11.3.min.js"></script>
<script src="/emojilib/js/config.js"></script>
<script src="/emojilib/js/util.js"></script>
<script src="/emojilib/js/jquery.emojiarea.js"></script>
<script src="/emojilib/js/emoji-picker.js"></script>
<script>
$(function() {
window.emojiPicker = new EmojiPicker({
emojiable_selector: '[data-emojiable=true]',
assetsPath: 'emojilib/img/',
popupButtonClasses: 'fa fa-smile-o'
});
window.emojiPicker.discover();
});
</script>
</body>
</html>
In the 'index.php' file, we have added a PHP file 'sendmail.php' at the top. This file contains code to send mail using the PHP mail() function. To send emojis or Unicode in the mail subject and body, we need to set the charset value 'UTF-8' in the header.
sendmail.php
<?php
$username = $_POST['name'];
$comment = $_POST['comment'];
$email = $_POST['email'];
$subject = $_POST['subject'];
$to = This email address is being protected from spambots. You need JavaScript enabled to view it. ';
if($email && $comment){
// To send HTML mail, the Content-type header must be set
$headers[] = 'MIME-Version: 1.0';
$headers[] = 'Content-type: text/html; charset=UTF-8';
// Additional headers
$headers[] = 'To: '.$to;
$headers[] = 'From: '.$email;
$mail = mail($to, $subject, $comment, implode("\r\n", $headers));
if ($mail) {
echo "<strong>Message sent!</strong>";
} else {
echo error_get_last()['message'];;
}
}
?>
So, this is how we send email with emojis in the mail subject and body with a few lines of code.
Related Articles
PHP code to send email using SMTPPHP Sending HTML form data to an Email
Retrieve Emails from Gmail using PHP IMAP
PHP reverse a string without predefined function
PHP random quote generator
PHP remove HTML and PHP tags from string
Import Excel File into MySQL using PHP
PHP array length
Import Excel File into MySQL Database using PHP
PHP String Contains
PHP remove last character from string
PHP Emoji Unicode Characters
How to store Emoji character in MySQL using PHP
Recover forgot password using PHP and MySQL
How to display PDF file in PHP from database
How to read CSV file in PHP and store in MySQL
Create And Download Word Document in PHP
PHP SplFileObject Standard Library
Simple File Upload Script in PHP