Bootstrap modal popup example on page load
In this article, you will learn step-by-step about Bootstrap Modal Popup and how to utilise it to create a newsletter subscription form.
Bootstrap is a strong front-end structure used to create advanced websites and applications. It is lightweight, easily customizable, and supports the major browsers and CSS compatibility fixes. It's open-source and free to utilise, yet it includes various HTML and CSS layouts for UI interface components, for example, buttons and frames. It supports JavaScript components, so one doesn't require knowledge of scripting. It comprises JavaScript components like tooltips, modal windows, alerts, and so on, to add functionalities without any problems.
A modal popup window is a child window that expects clients to interact with it before they can return to the parent application. So the modal box should provide a much better user experience. The Bootstrap model box is a perfect solution to add dialogues to your site for lightboxes, user notifications, alerts, or completely custom content, images, or video. It is built with HTML, CSS, and JavaScript. They're situated over all the other things in the document and eliminate look from the <body> with the goal that modal content scrolls instead. It only supports one modal window at a time. Nested modals aren't supported as we believe they provide poor user experiences. It definitely increases the usability of the website and decreases the load times.
Bootstrap Modal Popup Subscription Form
In the given example, we have created a newsletter subscription form using a bootstrap modal popup. First, we need to include the bootstrap JS and CSS files and the jQuery library. Then provide a unique id name to the modal popup div element, and write jQuery to show the modal popup window. The .modal('show') method of bootstrap is used for launching the modal window automatically on page load without clicking anything.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Bootstrap modal popup example on page load</title>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css" >
<script src="https://ajax.aspnetcdn.com/ajax/jQuery/jquery-3.3.1.min.js"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js"></script>
<script>
$(document).ready(function(){
$("#subscribe").modal('show');
});
</script>
</head>
<body>
<div id="subscribe" class="modal fade">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h3 class="modal-title">Subscribe to the newsletter</h3>
<button type="button" class="close" data-dismiss="modal">×</button>
</div>
<div class="modal-body">
<p>Subscribe to our newsletter to receive the latest news and updates in your inbox.</p>
<form action="subscription.html">
<div class="form-group">
<input type="text" class="form-control" placeholder="Enter your name">
</div>
<div class="form-group">
<input type="email" class="form-control" placeholder="Enter your email">
</div>
<button type="submit" class="btn btn-primary">Subscribe</button>
</form>
</div>
</div>
</div>
</div>
</body>
</html>
Output of the above code-
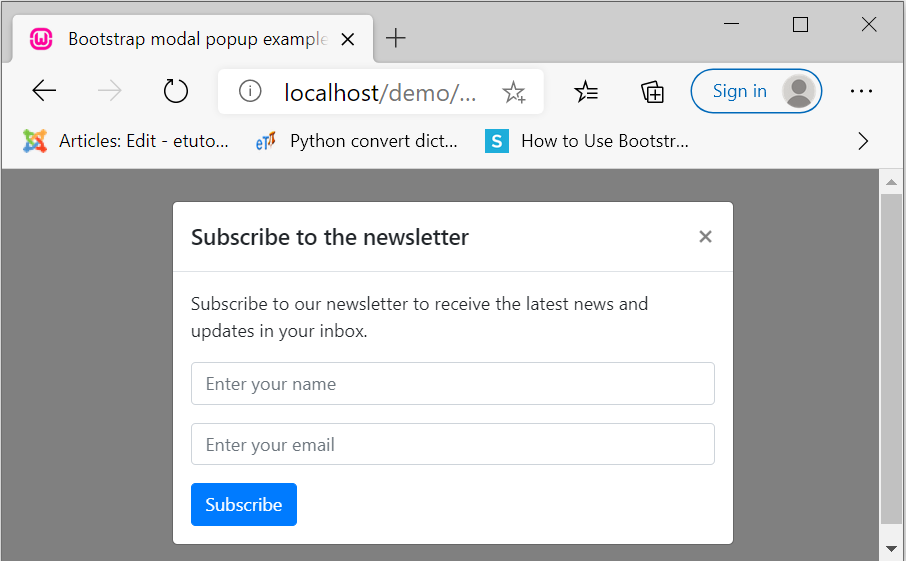
Related Articles
Scientific calculator JavaScriptPalindrome number in JavaScript
Star pattern program in JavaScript
Generate random numbers in JavaScript
Remove duplicates from array JavaScript
How to reverse a number in JavaScript
How to reverse string in JavaScript
JavaScript speech recognition example
jQuery sticky header on scroll
JavaScript display PDF in the browser using Ajax call
How to Retrieve Emails from Gmail using PHP IMAP
Retrieve Data From Database Without Page refresh using AJAX, PHP and Javascript
How to print specific part of a web page in javascript
How to store Emoji character in MySQL using PHP
How to display PDF file in PHP from database
jQuery loop over JSON result after AJAX Success
Dynamically Add/Delete HTML Table Rows Using Javascript
Submit a form data without page refresh using PHP, Ajax and Javascript
PHP Server Side Form Validation
How to add google reCAPTCHA v2 in registration form using PHP
Complete HTML Form Validation in PHP