How to insert image in database using PHP
In this post, you will learn how to insert an image into the database using the PHP programming language. In some applications, there may be a requirement to develop an image upload. The database is the preferred way to store data, and the file system is the best place to store files. For storing an image in a database, we can store the name or path of the image in the database and store the image as a file on the server. So that it can be easily accessed by the web server and delivered to the visitor.
PHP provides the easiest method for uploading and storing images in the MySQL database and saving the image in a particular location. First, let's create an HTML form that allows users to choose the image file they want to upload. The enctype='multipart/form-data' form attributes allow files to be sent through the post.
<form method='post' action='#' enctype='multipart/form-data'>
<div class="form-group">
<input type="file" name="image" >
</div>
<div class="form-group">
<input type='submit' name='submit' value='Upload' class="btn btn-primary">
</div>
</form>
The form looks something like this-
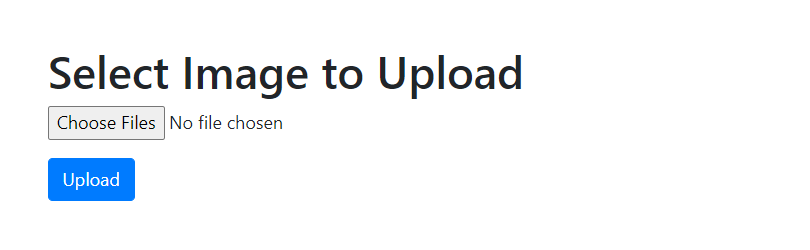
Next, create a database to store files. You can either copy and paste this CREATE statement into your database or use your existing one.
CREATE TABLE `image` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`file_name` varchar(255) COLLATE utf8_unicode_ci NOT NULL,
`uploaded_on` datetime NOT NULL,
`status` enum('1','0') COLLATE utf8_unicode_ci NOT NULL DEFAULT '1',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci;
Next, we have written the database connection code. Make sure to replace 'hostname', 'username', 'password', and 'database' with your database credentials and name.
$conn = mysqli_connect('hostname', 'username', 'password', 'database');
//Check for connection error
if($conn->connect_error){
die("Error in DB connection: ".$conn->connect_errno." : ".$conn->connect_error);
}
Next, we have written code to check the submitted image, valid file extension, and insert it into the database. The move_uploaded_file() function of PHP moves an uploaded file to a new destination. It returns TRUE on success and FALSE on failure.
if(isset($_POST['submit'])){
$filename = $_FILES['image']['name'];
// Select file type
$imageFileType = strtolower(pathinfo($filename,PATHINFO_EXTENSION));
// valid file extensions
$extensions_arr = array("jpg","jpeg","png","gif");
// Check extension
if( in_array($imageFileType,$extensions_arr) ){
// Upload files and store in database
if(move_uploaded_file($_FILES["image"]["tmp_name"],'upload/'.$filename)){
// Image db insert sql
$insert = "INSERT into image(file_name,uploaded_on,status) values('$filename',now(),1)";
if(mysqli_query($conn, $insert)){
echo 'Data inserted successfully';
}
else{
echo 'Error: '.mysqli_error($conn);
}
}else{
echo 'Error in uploading file - '.$_FILES['image']['name'].'
';
}
}
}
Complete Code: Insert image in database using PHP
Here, we have merged the above codes to upload an image to the database.
<?php
// database Connection
$conn = mysqli_connect('hostname', 'username', 'password', 'database');
// check for connection error
if($conn->connect_error){
die("Error in DB connection: ".$conn->connect_errno." : ".$conn->connect_error);
}
if(isset($_POST['submit'])){
$filename = $_FILES['image']['name'];
// Select file type
$imageFileType = strtolower(pathinfo($filename,PATHINFO_EXTENSION));
// valid file extensions
$extensions_arr = array("jpg","jpeg","png","gif");
// Check extension
if( in_array($imageFileType,$extensions_arr) ){
// Upload files and store in database
if(move_uploaded_file($_FILES["image"]["tmp_name"],'upload/'.$filename)){
// Image db insert sql
$insert = "INSERT into image(file_name,uploaded_on,status) values('$filename',now(),1)";
if(mysqli_query($conn, $insert)){
echo 'Data inserted successfully';
}
else{
echo 'Error: '.mysqli_error($conn);
}
}else{
echo 'Error in uploading file - '.$_FILES['image']['name'].'<br/>';
}
}
}
?>
<html>
<head>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js"></script>
</head>
<body>
<div class="container">
<h1>Select Image to Upload</h1>
<form method='post' action='#' enctype='multipart/form-data'>
<div class="form-group">
<input type="file" name="image" id="file" multiple>
</div>
<div class="form-group">
<input type='submit' name='submit' value='Upload' class="btn btn-primary">
</div>
</form>
</div>
</body>
</html>
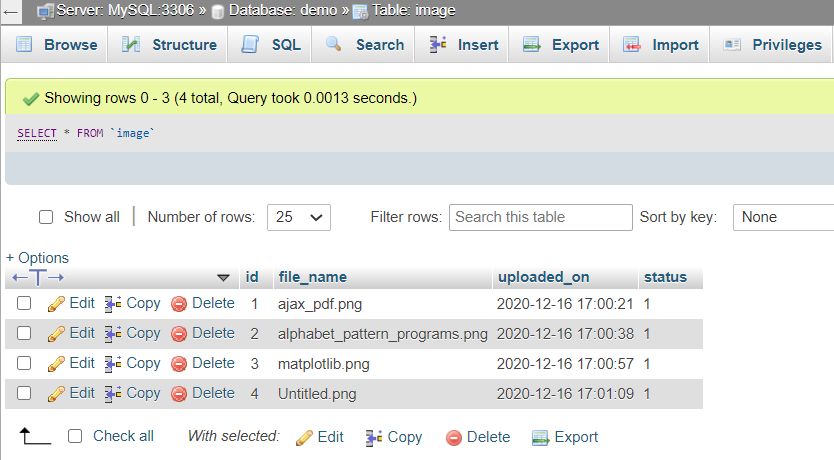
Related Articles
PHP reverse a string without predefined functionPHP random quote generator
PHP convert string into an array
PHP remove HTML and PHP tags from string
Import Excel File into MySQL using PHP
PHP array length
Import Excel File into MySQL Database using PHP
PHP String Contains
How to display PDF file in PHP from database
How to read CSV file in PHP and store in MySQL
Create And Download Word Document in PHP
PHP SplFileObject Standard Library
Simple File Upload Script in PHP
Sending form data to an email using PHP
Recover forgot password using PHP and MySQL
Php file based authentication
Simple PHP File Cache
How to get current directory, filename and code line number in PHP
Insert in database without page refresh PHP