Express js Middleware
Node.js gives us only one request handler function. Suppose we want to send large image files through node.js. This process will get complex in node.js. Express.js overcomes this problem. In express.js, we can call several request handler functions that can deal with small pieces of code rather than one request handler. These smaller request handler functions are called Middleware.
By using middleware, we can handle several tasks like, authenticating users, logging request, sending secure files, etc. Express.js middleware concepts are similar to the middleware of other languages, like Laravel of PHP, Django of Python, Rack middleware of Ruby.
Concept of Middleware
The following diagram demonstrates you with middleware concepts.
Request Handler without Middleware
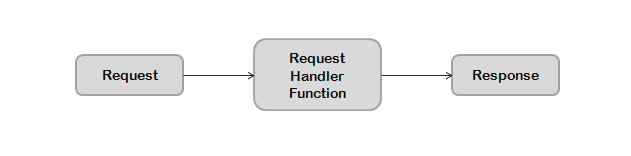
Request Handler with Middleware
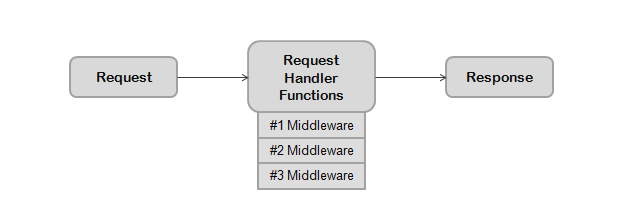
In the first diagram, the request is passed through only one request handler. This process will become more complex when we deal with large applications. This complexity is overcome by middleware. As we can see in the second diagram, the request passes through an array of request handler functions.
Syntax of Middleware
function(request, response, next)
In the above request handler function, the third argument 'next' is itself a function. When this is called, the express will go on to the next function in the stack. This function call inside a route handler invokes the next route handler only. If we will define a middleware next to it, then it is skipped. So we must declare middleware above all route handlers.
Middleware Example
This is the following simple middleware example.
var express = require('express');
var app = express();
app.use(function (req, res, next) {
console.log('Time:', Date.now());
next();
});
app.get("/", function(req, res, next){
response.send("Hello World!");
next();
});
app.use('/', function(req, res){
console.log('End');
});
app.listen(3000, function(){
console.log('Server is running at http://localhost:3000');
});