PHP7 Namespaces
Namespace is an advanced PHP concept, it officially released with PHP 5.3. It is introduced to avoid the problem of accidental class duplication or we can say that it reduces class collision and confusion.
A namespace class contains only valid PHP code. It affects only classes, interfaces, functions and constants. The namespace names are case-insensitive.
Create Namespace
To define a class with the namespace, simply add the keyword namespace at the top of the code file with one exception of declare keyword.
Namespace Example
<?php
namespace Flipkart;
class Sell{
public function product(){
return 'Flipkart Product Names';
}
}
namespace Amazon;
class Sell{
public function product(){
return 'Amazon Product Names';
}
}
?>
The concept of a namespace is very clear from the above code. There are two namespaces 'Flipkart' and 'Amazon', and both has same class and method names, i.e. Sell class and product() method. Let's think, what will happen when we call the both classes of same name in the same file without namespace, obviously it returns fatal error. So namespace is used to avoid collisions between classes, interfaces, functions and constants.
Sub Namespaces
We can also declare a single namespace with hierarchy of namespace names.
<?php
namespace Module/Entity;
?>
How to use PHP namespaces
Suppose, we have a directory name 'Module' and it has a subdirectory 'Entity'. It contains three PHP files as shown below -
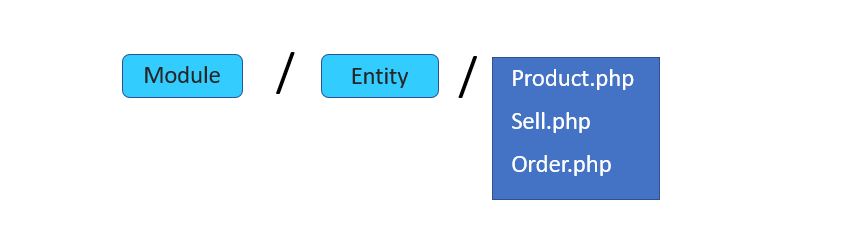
To use a class in an external namespace, use statement is used. It is prepended to the namespace.
<?php
use Module/Entity;
?>
We can also specify the class name with use statement, like
<?php
use Module/Entity/Product;
?>
PHP7 introduces new feature group use, which is basically used to specify more classes.
<?php
use Module/Entity/ {
Product,
Sell,
Order
};
$product = new Product();
$sell = new Sell();
$order = new Order();
?>
__NAMESPACE__
It is a constant the returns the current namespace in string.
<?php
namespace Flipkart;
echo '"', __NAMESPACE__, '"';
?>
In the global namespace, it returns an empty string.
<?php
echo '"', __NAMESPACE__, '"';
?>