Fibonacci series in C using function
In this program, you'll learn to display the fibonacci sequence using function in the C programming language.
The Fibonacci series is a sequence of numbers in which the next number is the sum of the previous two numbers. The Fibonacci series was well-known hundreds of years earlier. The "Fibonacci" name came from the nickname "Bonacci".
We can easily remember the Fibonacci sequence by using November 23rd as Fibonacci Day. As November 23rd has the digits "1, 1, 2, 3", which is part of the sequence.
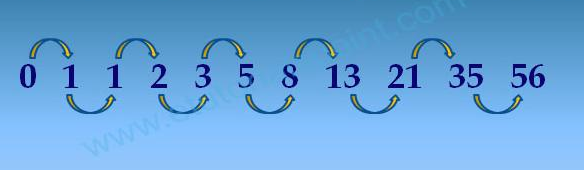
In the above image, the first two numbers are 0 and 1. So, according to the Fibonacci rule, the third number is 1 (sum of 0 and 1). The fourth number is 2, and so on.
0 + 1 = 1 // 0, 1, 1
1 + 1 = 2 // 0, 1, 1, 2
1 + 2 = 3 // 0, 1, 1, 2, 3
2 + 3 = 5 // 0, 1, 1, 2, 3, 5
0 ,1 , 1, 2, 3, 5, 8, 13, 21, 34....
Fibonacci series in C using function
In the given C program, we define a function fibonacciSeries(), initialise n1 to 0 and n2 to 1, and display it to the console. Next, we add n1 and n2, assign them to n3, and display the value of n3. We use the for loop to keep displaying the Fibonacci series until the limit entered by the user.
#include<stdio.h>
void fibonacciSeries(int);
int main()
{
int n;
printf("How many number of series? :\n");
scanf("%d", &n);
// calling function
fibonacciSeries(n);
return 0;
}
void fibonacciSeries(int num)
{
int n1 = 0, n2 = 1, n3, count;
printf("\nFibonacci Series: \n");
printf("1. %d\n2. %d\n", n1, n2);
for(count = 3; count <= num; count++)
{
n3 = n1 + n2;
printf("%d. %d\n", count, n3);
n1 = n2;
n2 = n3;
}
}
Fibonacci series in C using Recursive Function
In the given C program, we call the recursion function to get the Fibonacci series. The function fibonacciSeries() is called recursively until we get the result. In the function, we first check if the number n is greater than 0. If yes, we recursively call fibonacciSeries() with the values n-1 and n-2.
// C program to print Fibonacci series
#include<stdio>
// Recursive function
void fibonacciSeries(int n){
static int n1=0,n2=1,n3;
if(n>0){
n3 = n1 + n2;
n1 = n2;
n2 = n3;
printf("%d ",n3);
fibonacciSeries(n-1);
}
}
int main(){
int n;
printf("How many number of series? : ");
scanf("%d",&n);
printf("Fibonacci Series: ");
printf("%d %d ",0,1);
//Call Function
fibonacciSeries(n-2);
return 0;
}
Output of the above code:
How many number of series? : 15
Fibonacci Series: 0 1 1 2 3 5 8 13 21 34 55 89 144 233 377
Related Articles
Average of two numbers in CSwapping of two numbers in C using pointers
Armstrong number in C using function
Binary to decimal C program
Sum of array elements in C
Random number generator in C
Factorial program in c using while loop
Student mark sheet program in C
C program to sort names in alphabetical order
C program to find largest number in an array
Print first 10 natural numbers using while loop in C
Simple calculator program in C
C program for simple interest
Swap two numbers without using third variable in C
Radix sort program in C
Bit stuffing program in C
Bubble sort program in C
Decimal to hexadecimal in C
Hexadecimal to decimal in C
Quick sort program in C